Pythonというプログラミング言語を使えば、自動で毎朝励ましの言葉を届けるチャットボットを作れます。プログラミング初心者の方でも簡単に取り組めるよう丁寧に解説します。
忙しい毎日の中で、ポジティブな言葉は大きな励みになります。ぜひ自分だけの「ほめるチャットボット」を作って、日々の活力にしてください。
ほめるチャットボットの作り方
Pythonの環境を準備する
コマンドプロンプトを開いて、以下のコマンドで必要なライブラリをインストールします。
pip install requests beautifulsoup4 schedule pytz
LINE Notifyを使ってメッセージを送信
LINE NotifyでAPIキーを取得し、以下のコードでメッセージを送ります。
import requests
class LineNotify:
def __init__(self, token):
self.token = token
def send_message(self, message):
headers = {'Authorization': f'Bearer {self.token}'}
payload = {'message': message}
requests.post('https://notify-api.line.me/api/notify', headers=headers, params=payload)
# 使用例
line = LineNotify('YOUR_LINE_NOTIFY_TOKEN')
line.send_message('素晴らしい一日ですね!')
天気情報を取得して活用する
WeatherAPIを使って天気情報を取得し、メッセージに反映します。
import requests
class WeatherAPI:
def __init__(self, api_key):
self.api_key = api_key
def get_weather(self, location):
params = {'key': self.api_key, 'q': location}
response = requests.get('http://api.weatherapi.com/v1/current.json', params=params)
if response.status_code == 200:
data = response.json()
return f"{data['current']['condition']['text']}, 気温: {data['current']['temp_c']}℃"
else:
return '天気情報の取得に失敗しました。'
会話履歴をSQLiteに保存する
ユーザーとのやり取りを保存するためにSQLiteを利用します。
import sqlite3
class ChatHistoryDB:
def __init__(self, db_name='chat_history.db'):
self.conn = sqlite3.connect(db_name)
self.cursor = self.conn.cursor()
self._create_table()
def _create_table(self):
self.cursor.execute('''
CREATE TABLE IF NOT EXISTS chat_history (
id INTEGER PRIMARY KEY AUTOINCREMENT,
user_input TEXT,
bot_response TEXT,
timestamp DATETIME DEFAULT CURRENT_TIMESTAMP
)
''')
self.conn.commit()
def save_chat(self, user_input, bot_response):
self.cursor.execute('''
INSERT INTO chat_history (user_input, bot_response) VALUES (?, ?)
''', (user_input, bot_response))
self.conn.commit()
タスクスケジューラーを使って自動化
毎日決まった時間に自動的に実行します。
- cronの設定例(Linux/Mac):
0 8 * * * /usr/bin/python3 /path/to/your_script.py
- タスクスケジューラーの設定(Windows):
タスクスケジューラーを開き、スクリプトを指定し、毎朝実行するよう設定します。
完成したチャットボットの完全なコード
すべての機能を統合したコード例はこちらです。
import requests
import random
import sqlite3
import pytz
from datetime import datetime
class LineNotify:
def __init__(self, token):
self.token = token
def send_message(self, message):
headers = {'Authorization': f'Bearer {self.token}'}
payload = {'message': message}
requests.post('https://notify-api.line.me/api/notify', headers=headers, params=payload)
class WeatherAPI:
def __init__(self, api_key):
self.api_key = api_key
def get_weather(self, location):
params = {'key': self.api_key, 'q': location}
response = requests.get('http://api.weatherapi.com/v1/current.json', params=params)
if response.status_code == 200:
data = response.json()
return f"{data['current']['condition']['text']}, {data['current']['temp_c']}℃"
else:
return '天気情報の取得に失敗しました。'
class ChatHistoryDB:
def __init__(self, db_name='chat_history.db'):
self.conn = sqlite3.connect(db_name)
self.cursor = self.conn.cursor()
self.cursor.execute('''CREATE TABLE IF NOT EXISTS chat_history (
id INTEGER PRIMARY KEY,
user_input TEXT,
bot_response TEXT,
timestamp DATETIME DEFAULT CURRENT_TIMESTAMP)''')
def save_chat(self, user_input, bot_response):
self.cursor.execute('INSERT INTO chat_history (user_input, bot_response) VALUES (?, ?)', (user_input, bot_response))
self.conn.commit()
class HometeChatbot:
def __init__(self, line_notify_token, weather_api_key):
self.line_notify = LineNotify(line_notify_token)
self.weather_api = WeatherAPI(weather_api_key)
self.chat_db = ChatHistoryDB()
def send_homete_message(self):
messages = ["素晴らしい一日ですね!", "君は本当にすごい!", "今日も最高だね!"]
weather = self.weather_api.get_weather('Tokyo')
message = random.choice(messages) + f'\n東京の天気:{weather}'
self.line_notify.send_message(message)
def start(self):
self.send_homete_message()
user_input = input('あなた:')
response = "頑張っていますね!"
print(f'チャットボット:{response}')
self.chat_db.save_chat(user_input, response)
if __name__ == "__main__":
chatbot = HometeChatbot('YOUR_LINE_NOTIFY_TOKEN', 'YOUR_WEATHER_API_KEY')
chatbot.start()
最後に
Pythonで気軽にAIから励ましをもらい、充実した毎日を送りましょう!ぜひ、自分だけのオリジナルチャットボットを作成してみてください。
この記事でPythonに可能性を感じた、Pythonを学んでみたいと思ったという方はぜひ、Python入門講座のリンクへ飛んでみてくださいね。
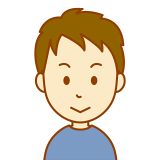
白川秋
ではでは、参考までに。
コメント